Many project-specific tasks inside FirstSpirit are done by scripts, so that they run automatically and don’t have to be done manually by the editors. As long as the code of a script is not very long a Beanshell-script inside FirstSpirit is the best solution.
But if the code has “countless” lines it makes sense to use a modul. With the use of a module code can be split into classes, which are able to interact. This makes long code maintainable and follows the paradigm of object-oriented programming.
Furthermore the Beanshell-interpreter isn’t needed when modules are used instead of scripts. An interpreter always needs time to interpret and execute code. So not needing an interpreter means conserving time.
This blogposting explains how a module, which contains a simple Executable-class, can be created and how this could be used inside a FirstSpirit project.
Therefore the following steps are needed:
1. Create the module
2. Install the module at the FirstSpirit server
3. Create the script
4. Try it!
Create the module
Get the sourcecode
The complete sourcecode of the described example can be downloaded via a GitHub-Repository, which can be found here.
Among others this sourcecode includes the module.xml, which is used to describe the module.
<?xml version="1.0" encoding="UTF-8"?>
<module>
<name>${project.artifactId}</name>
<version>${project.version}</version>
<description>${project.artifactId}</description>
<vendor>com.espirit.moddev.knowledgebase.simpleExecutableExample</vendor>
<components>
<public>
<name>SimpleExecutableExample</name>
<class>com.espirit.moddev.knowledgebase.simpleExecutableExample.${project.artifactId}Executable</class>
</public>
</components>
<resources>
<resource scope="module">lib/${project.artifactId}-${project.version}.jar</resource>
</resources>
</module>
In this case the module.xml shows the name, the version, a description and information of the vendor of the to be created module. As the only inserted component the Executable-class is listed. Further infomation can be found in the Manual for Developers (Components). (not yet available in English)
The package “com.espirit.moddev.knowledgebase.simpleExecutableExample" contains this Executable-class:
public class SimpleExecutableExampleExecutable implements Executable { public Object execute(Map<String, Object> params) throws ExecutionException {
ScriptContext context = (ScriptContext) params.get("context");
OperationAgent operationAgent = context.requireSpecialist(OperationAgent.TYPE);
RequestOperation operation = operationAgent.getOperation(RequestOperation.TYPE);
operation.perform("Hello World!");
return true;
}
public Object execute(Map<String, Object> context, Writer out, Writer err) throws ExecutionException {
return execute(context);
}
}
It is very important that this class implements the Executable-interface with its two execute-methods. Without implementing this interface, the module wouldn't work.
An explanation about the RequestOperation can be find here.
To make this class available in the used FirstSpirit project, a module is needed, which first has to be built.
Build the module
Next to the sourcecode the GitHub-Repository contains a "pom.xml". With using Maven it is possible to build a new module based on this file.
(If you first need to install Maven, follow the instructions here.)
For a successful compilation of the sourcecode it is necessary to install the "fs-access.jar"-file of the FirstSpirit-server in the local Maven-Repository. (The local Maven-Repository was created automatically while the Maven-installation. It can be find under ${user.home}/.m2/repository.)The "fs-access.jar"-file is located under the directory <FS-Server-Folder>/data/fslib.
The following command will trigger the installation:
mvn install:install-file -Dfile=<path-to-acces.jar> -DgroupId=de.espirit.firstspirit -DartifactId=fs-access -Dversion=<fs version e.g. '5.0.0'> -Dpackaging=jar
Within this command the path to the "fs-acces.jar"-file has to be replaced.
After the installation of the "fs-access.jar"-file to the Maven-Repository the new module can be build with this command:
mvn package -Dci.version=<version> -Dfirstspirit.version=<fs-version>
Within this command the "version" and the the "fs-version" has to be replaced. The "fs-version" has to be the same as the "Dversion" in the previous command.
At the directory of the pom.xml a new subfolder "target" will be created. This folder contains the new module, which has to be installed at the FirstSpirit-server.
Install the module
After opening the server properties the module can be installed inside the “Modules”-tab by clicking the button “Install”. This brings up a selection-dialog, by what the fsm-file can be chosen from the local file system. If the installation is successful, the module will be added to the displayed list of installed modules. After the installation the files of the module are available for all projects on the FirstSpirit server.
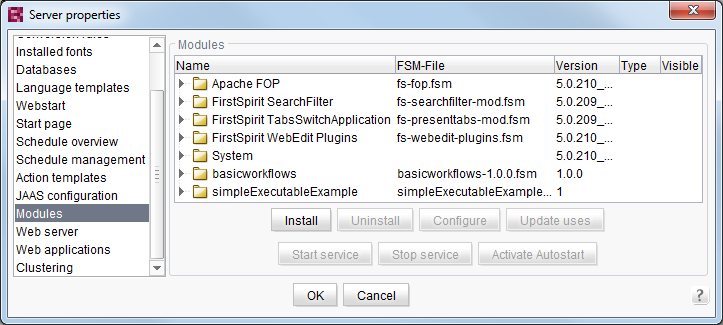
Create the script
To use the code of the module inside a FirstSpirit project a script is needed. This script has just two lines, which contain the command to call the class inside the module.
#!executable-class
com.espirit.moddev.knowledgebase.simpleExecutableExample.SimpleExecutableExampleExecutable
To execute the script from inside the context menu, it has to have the type “Context menu”.
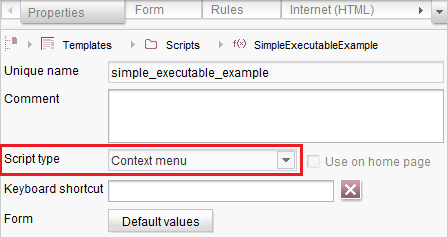
Try it!
Now all steps are done to start the script from inside the context menu. Executing the script should pop up a dialog, which contains the text “Hello World!”.
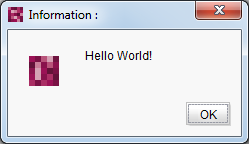